Q:
How to apply filters to images using Python and OpenCV?
belongs to collection: Python Exercises, Practice Questions and Solutions
Python Exercises, Practice Questions and Solutions
- Write a program in Python to use the string join operation to create a string that contains a colon as a separator
- Write a program in Python to replace a, b with 1, 3 respectively in a string
- Write a program in Python to encode and decode the following string
- Write a program in Python to read content from one file and write in another file.
- Write a program in Python to create a dictionary from the given sequence of keys and value provided by the user
- Write a list comprehension that returns all the pairs in a dictionary whose associated values are greater than zero
- Write a program in Python to keep count of this given list starting from 100
- Write a program in Python to calculate the value of the following expression by using lambda function
- Write a program in Python to calculate the Fahrenheit of the following Celsius list by using Lambda function
- Write a program in Python to filter the given list if the given expression evaluates to true
- Write a Python program to count the occurrences of each word in a given sentence
- Write a program to calculate simple interest in Python
- Write a python program to calculate total marks percentage and grade of a student
- Write a python program to find the area of a rectangle in python
- Write a program to find the largest of three numbers in Python
- How do you transpose a matrix using list comprehension in Python?
- How do you make a simple calculator in Python?
- How do you find the prime factor of a number in Python?
- Write a program to read two numbers and print their quotient and remainder in Python
- Write a python program to sort words in alphabetical order
- Write a python program to sum all the numbers in a list
- How to apply filters to images using Python and OpenCV?
- Write a python program to input week number and print week day
- Write a python program to map two lists into a dictionary
- Python program to multiply two numbers
- Write a python program to remove last element from list
- How do you find the GCF in Python?
- How to multiply all elements in list Python?
- How do you count consonants in a string in python?
- How to read JSON from URL requests in python?
- Write a node.js program for making external http calls
Averaging Filter
The Averaging Filter technique takes the average of all the pixels under the kernel area and replaces the central element. The function cv2.blur() and cv2.boxFilter() can be used to perform the averaging filter. Both functions smooth an image using the kernel.
Syntax of cv2.blur()
cv2.blur(image, ksize)
Here, the image is the image source and ksize is the size of blurring kernel.
Syntax of cv2.boxFilter()
cv2.boxFilter(src, dst, depth, ksize, anchor, normalize, bordertype)
Here, the src is the image source, dst is the destination image of the same size, depth denotes the output image depth. The anchor denotes the anchor points. By default, it is at kernel point (cordinate (-1,1)). The ksize is the size of blurring kernel and normalize is the flag which specifies whether the kernel should be normalized or not. The bordertype is an integer object represents the type of the border used.
Example of Averaging Filter
Gaussian Filter
The OpenCV Gaussian filtering provides cv2.GaussianBlur() method to blur an image by using Gaussian Kernel. Each pixel in an image gets multiplied by Gaussian Kernel. It means, a Gaussian Kernel is a square array of pixels.
Syntax of Gaussian Filter
cv2.GaussianBlur(src, ksize, sigma_x, dst, sigma_y, border_type)
src - the input image,
ksize - Gaussian kernel size (width and height), the width and height can have different values and must be positive and odd,
sigma_x - Gaussian kernel standard deviation along X-axis,
dst - output image,
sigma_y - Gaussian kernel standard deviation along Y-axis,
boader_type - image boundaries.
Example of Gaussian
Median Filtering
Python OpenCV provides the cv2.medianBlur() function to blur the image with a median kernel. This is a non-linear filtering technique. It is highly effective in removing salt-and-pepper noise. This takes a median of all the pixels under the kernel area and replaces the central component with this median value. Since we are taking a middle, the output image will have no new pixel esteems other than that in the input image.
Syntax of Median Filter
cv2.medianBlur(image, ksize)
Here, the image is representing the image for operation, the ksize is a size object representing the size of the kernel.
Example of Median Filter
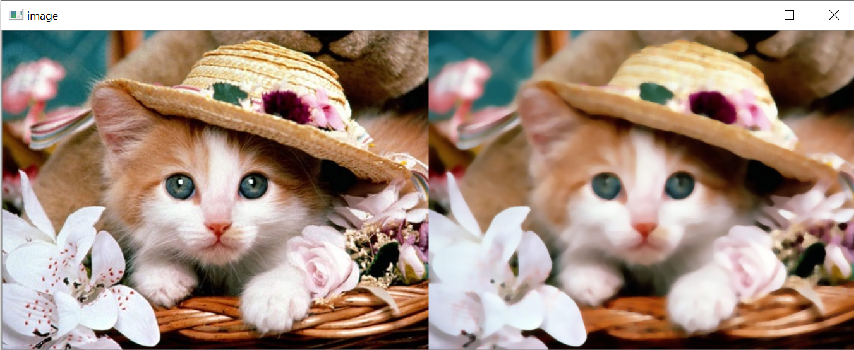
need an explanation for this answer? contact us directly to get an explanation for this answer