Q:
How do you make a simple calculator in Python?
belongs to collection: Python Exercises, Practice Questions and Solutions
Python Exercises, Practice Questions and Solutions
- Write a program in Python to use the string join operation to create a string that contains a colon as a separator
- Write a program in Python to replace a, b with 1, 3 respectively in a string
- Write a program in Python to encode and decode the following string
- Write a program in Python to read content from one file and write in another file.
- Write a program in Python to create a dictionary from the given sequence of keys and value provided by the user
- Write a list comprehension that returns all the pairs in a dictionary whose associated values are greater than zero
- Write a program in Python to keep count of this given list starting from 100
- Write a program in Python to calculate the value of the following expression by using lambda function
- Write a program in Python to calculate the Fahrenheit of the following Celsius list by using Lambda function
- Write a program in Python to filter the given list if the given expression evaluates to true
- Write a Python program to count the occurrences of each word in a given sentence
- Write a program to calculate simple interest in Python
- Write a python program to calculate total marks percentage and grade of a student
- Write a python program to find the area of a rectangle in python
- Write a program to find the largest of three numbers in Python
- How do you transpose a matrix using list comprehension in Python?
- How do you make a simple calculator in Python?
- How do you find the prime factor of a number in Python?
- Write a program to read two numbers and print their quotient and remainder in Python
- Write a python program to sort words in alphabetical order
- Write a python program to sum all the numbers in a list
- How to apply filters to images using Python and OpenCV?
- Write a python program to input week number and print week day
- Write a python program to map two lists into a dictionary
- Python program to multiply two numbers
- Write a python program to remove last element from list
- How do you find the GCF in Python?
- How to multiply all elements in list Python?
- How do you count consonants in a string in python?
- How to read JSON from URL requests in python?
- Write a node.js program for making external http calls
Simple Calculator Program in Python
Sample output of the above program -
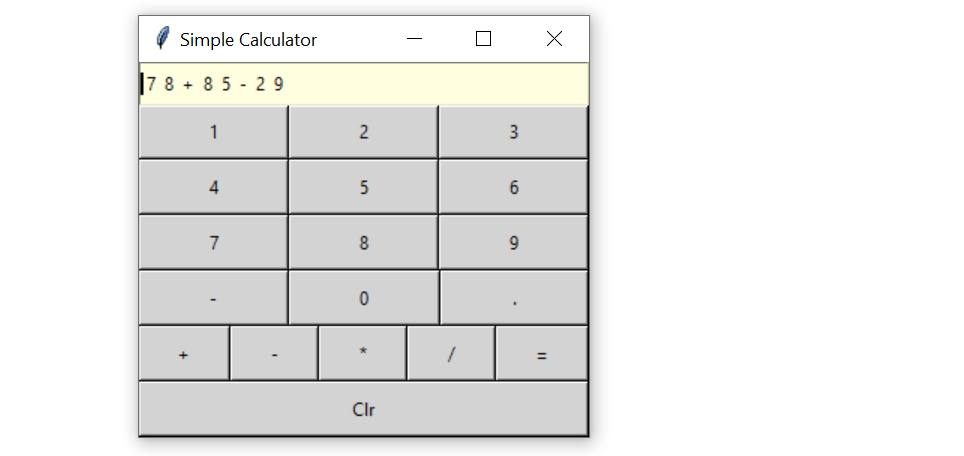
need an explanation for this answer? contact us directly to get an explanation for this answer