Q:
Implementations of FCFS scheduling algorithm using C++
belongs to collection: C++ programs on various topics
C++ programs on various topics
- C++ - Employee Management Program through Binary File Handling
- C++ program for flight booking system
- C++ program - Polymorphism implementation using Virtual functions.
- C++ program for Constructor and Destructor Declaration, Definition
- C++ Class Exercise - Read and Print Class, Students Details using Two Classes
- C++ Class Exercise - Read and Print House details along with Room details.
- C++ program to demonstrate example of Templates.
- C++ - Read Characters from One file and Write them in Toggle Case in Other using C++ file stream.
- C++ program to write and read time in/from binary file using fstream
- C++ program to write and read an object in/from a binary file
- C++ program to print the size of different types of pointers along with values and addresses
- C++ - Print the string character by character using pointer
- C++ program to declare, read and print dynamic integer array
- C++ program to declare an integer variable dynamically and print its memory address
- C++ - Initialization of Array of Objects with Parameterized Constructor in C++ program.
- C++ - program for Nested Structure (Structure with in Structure).
- C++ - program for Array of Structure.
- C++ program to demonstrate calling of private member functions inside public member function.
- C++ program to demonstrate use of protected data members in inheritance.
- C++ program to demonstrate calling of private member functions inside public member function.
- C++ program to demonstrate use of protected data members in inheritance.
- C++ program to access public data member inside main using object name.
- C++ program to declare, define and access public static data member.
- C++ program to print ASCII value of a character.
- C++ program to print the maximum possible time using six of nine given single digits
- Print Reverse Triangle Bridge Pattern for Characters in C++
- Sort an array in ascending order using sort() function in C++ STL
- Sort an array in descending order using sort() function in C++ STL
- Binary Search in C++ using Standard Template Library (STL) function binary_search()
- Stack program using C++ Standard Template Library (STL)
- C++ program to find the integers which come odd number of times in an array
- Vectors in C++ Standard Template Library (STL)
- C++ program to Find Nth element of the X-OR sequence
- Implementations of FCFS scheduling algorithm using C++
- Implementation of Shortest Job First (SJF) Non-Preemptive CPU scheduling algorithm using C++
- Implementation of Shortest Job First (SJF) Preemptive CPU scheduling algorithm using C++
- Implementation of Priority Scheduling (Pre-emptive) algorithm using C++
- Implementation of Priority scheduling (Non Pre-emptive) algorithm using C++
- Implementation of Round Robin CPU Scheduling algorithm using C++
- Jump Search Implementation using C++
- How to check if a number is power of 2 or not in C++ (different methods)?
- Print character through ASCII value using cout in C++
- C++ program to find and print first uppercase character of a string
- C++ program to declare, read and print dynamic integer array
- Count the created objects using static member function in C++
- C++ program to print the size of different types of pointers along with values and addresses
- C++ program to declare an integer variable dynamically and print its memory address
- new and delete operators in C++ with printing values through constructor and destructor
- C++ program to generate random password
- C++ program to print all possible subset of a set
- C++ program to print all possible subset of a set
- Find total Number of bits required to represent a number in binary in C++
- Find next and previous power of two of a given number in C++
- Create an object of a class inside another class declaration in C++
- Example of private member function in C++
- Local Class with Example in C++
- Structure with private members in C++
- Const Member Functions in C++
- Demonstrate Example of public data members in C++
- Dynamic Initialization of Objects in C++
- Create a class Point having X and Y Axis with getter and setter functions in C++
- Passing an object to a Non-Member function in C++
- Set values of data members using default, parameterized and copy constructor in C++
- Accessing Member Function by pointer in C++
- Access the address of an object using \'this\' pointer in C++
- Create a class with public data members only in C++
- Sum of all the elements in an array divisible by a given number K
- Find the roots of quadratic equation in C++
- C++ program to find Sum of cubes of first N Even numbers
- Sorting a structure in C++
- C++ program to multiply two numbers without using multiplication operator
- C++ program to find LCM of two numbers
- C++ program Input list of candidates and find winner of the Election based on received votes
- C++ program of Airline Seat Reservation Problem
- C++ program to Convert Roman Number to Integer Number
- C++ program to find presence of a number X in the array recursively
- C++ program to print all subsequences of a String
- C++ program to find number of BSTs with N nodes (Catalan numbers)
- C++ program to find first occurrence of a Number Using Recursion in an array
- C++ program to find Last occurrence of a Number using Recursion in an array
- C++ program to obtain Multiplication recursively
- C++ program to check whether a given Binary Search Tree is balanced or not?
- Append Last N Nodes to First in the Linked List using C++ program
- Eliminate duplicates from Linked List using C++ program
- Find a Node in Linked List using C++ program
- Merge sort for single linked lists
- C++ program to find Union of two single Linked Lists
- Find intersection of two linked lists using C++ program
- Representing System of Linear Equations using Matrix
- iswlower() function in C++
- iswupper() function in C++
- C++ program to print the left Rotation of the array
- C++ program to check if number is power of 2 using Bitwise operator
- Implement Stack using Linked List in C++
- Delete the middle node of a Linked List in C++
- Delete keys in a Linked list using C++ program
- Reverse a Linked List in groups of given size using C++ program
- Pair wise swap elements in a linked list using C++ program
- Convert a given binary Tree to Doubly Linked List (DLL)
- Modify contents of Linked List using C++ program
- Delete N nodes after M nodes of a linked list using C++ program
- Clone a linked list with next and random pointer using C++ program
- Topological sort implementation using C++ program
- C++ program to determine the color of chess square
- C++ program to find the power of a number using loop
- C++ program to extract and print digits in reverse order of a number
- C++ program to print all Even and Odd numbers from 1 to N
- C++ | Create class methods
- C++ | Create multiple objects of a class
- C++ | Create an object of a class and access class attributes
- C++ program | Different ways to print array elements
- C++ template program with arrays
- C++ | Define a class method outside the class definition
- C++ | Assign values to the private data members without using constructor
- C++ | Create an empty class (a class without data members and member functions)
- C++ | Create a class with setter and getter methods
- C++ tellg(), seekg() and seekp() Example
- C++ program for Banking Management System using Class
FIRST COME FIRST SERVED (FCFS) Algorithm
As the name suggests in this algorithm process are schedule according to their arrival time, the process that comes first will be scheduled first and it will be in CPU until it completes it burst time.
C++ Program for FCFS Scheduling
Output
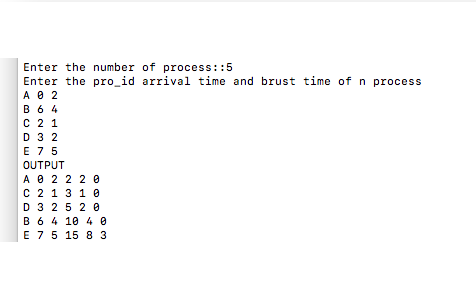
need an explanation for this answer? contact us directly to get an explanation for this answer