Explanation
In this program, we need to create an array and print the elements present in the array.
Arrays are the special variable that stores multiple values under the same name. A contiguous memory will be allocated to store elements. Elements of the array can be accessed through their indexes.
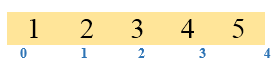
Here, 1, 2, 3, 4 and 5 represent the elements of the array. These elements can be accessed through their corresponding indexes, i.e., 0, 1, 2, 3 and 4.
Algorithm
- Declare and initialize an array.
- Loop through the array by incrementing the value of i.
- Finally, print out each element of the array.
Input:
arr = [1, 2, 3, 4, 5]
Output:
Elements of given array: 1 2 3 4 5
Python
Output:
C
Output:
JAVA
Output:
C#
Output:
PHP
Output: