Explanation
In this program, we will create a doubly linked list and sort nodes of the list in ascending order.
- Original List:
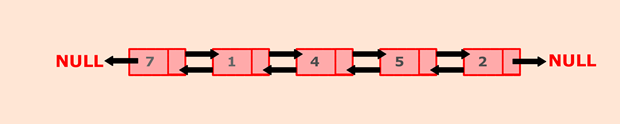
-
Sorted List:
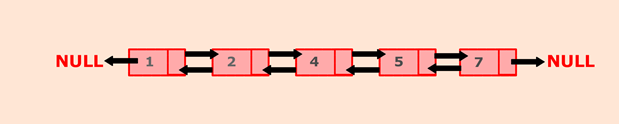
-
To accomplish this, we maintain two pointers: current and index. Initially, current point to head node and index will point to node next to current. Traverse through the list till current points to null, by comparing current's data with index's data. If the current's data is greater than the index's data, then swap data between them. In above example, current will initially point to 7, and the index will point to 1. Since, 7 is greater than 1, swap the data. Continue this process till the entire list is sorted in ascending order.
Algorithm
- Define a Node class which represents a node in the list. It will have three properties: data, previous which will point to the previous node and next which will point to the next node.
- Define another class for creating a doubly linked list, and it has two nodes: head and tail. Initially, head and tail will point to null.
- addNode() will add node to the list:
- It first checks whether the head is null, then it will insert the node as the head.
- Both head and tail will point to a newly added node.
- Head's previous pointer will point to null and tail's next pointer will point to null.
- If the head is not null, the new node will be inserted at the end of the list such that new node's previous pointer will point to tail.
- The new node will become the new tail. Tail's next pointer will point to null.
- sortList() will sort nodes of the list in ascending order.
- Define a node current which will point to head.
- Define another node index which will point to node next to current.
- Compare data of current and index node. If the current's data is greater than the index's data, then swap the data between them.
- Current will point to current.next and index will point to index.next.
- Continue this process till the entire list is sorted.
- display() will show all the nodes present in the list.
- Define a new node 'current' that will point to the head.
- Print current.data till current points to null.
- Current will point to the next node in the list in each iteration.
-
Input:
#Add nodes to the list
dList.addNode(7);
dList.addNode(1);
dList.addNode(4);
dList.addNode(5);
dList.addNode(2);
Output:
Original list: 7 1 4 5 2
Sorted list: 1 2 4 5 7
Python
Output:
C
Output:
JAVA
Output:
C#
Output:
PHP
Output: