Explanation
In this program, we will find out the smallest node in the given binary tree. We first define variable min that will hold root's data. Then, we traverse through left sub-tree to find the smallest node in left subtree. Compare it with min and store minimum of two in variable min. Then, we traverse through right subtree to find smallest node and compare it with min. At the end, min will have the smallest node.
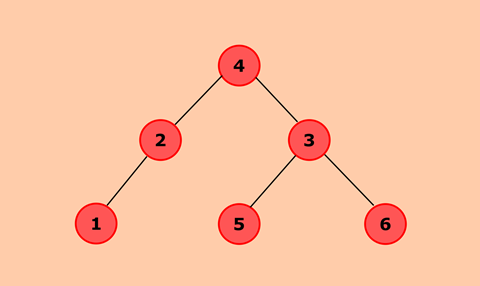
Above diagram represents a binary tree. Initially, min will hold 4. Recursive through left subtree.
min = 4, leftMin = 2 => (2 < 4) then min = 2
min = 2, leftMin = 1 => (1 < 2) then min = 1
Recursive through right subtree.
min = 1, rightMin = 3 => (1 < 3) then min = 1
Recurse in left subtree of 5
min = 1, leftMin = 5 => (1 < 5) then min = 1
Recurse in right subtree of 3
min = 1, rightMin = 6 => (1 < 6) then min = 1
So, smallest node in above binary tree is 1.
Algorithm
- Define Node class which has three attributes namely: data, left and right. Here, left represents the left child of the node and right represents the right child of the node.
- When a node is created, data will pass to data attribute of node and both left and right will be set to null.
- Define another class which has an attribute root.
- Root represent root node of the tree and initialize it to null.
- smallestElement() will find out the smallest node in binary tree:
- It checks whether root is null, which means tree is empty.
- If tree is not empty, define a variable min that will store temp's data.
- Find out the minimum node in left subtree by calling smallestElement() recursively. Store that value in leftMin. Compare the value of min with leftMin and store the minimum of two to min.
- Find out the minimum node in right subtree by calling smallestElement() recursively. Store that value in rightMin. Compare the value of min with rightMin and store the maximum of two to min.
- At the end, min will hold the smallest node in the binary tree.
Output:
Smallest element in the binary tree: 1
Python
Output:
C
Output:
JAVA
Output:
C#
Output:
PHP
Output: