#include <stdio.h>
int main()
{
int lines=15;
int i,j,k,l;
int space=0;
for(i=1;i<=lines;i++){// this loop is use to print lines
for(j=1;j<=space;j++){// this loop is used to print the space
printf(" ");
}
for(j=1;j<=lines;j++){// this loop is used to print numbers in a line
if(j<=(lines-i))
printf("%d",j);
else
printf("*");
}
j--;
while(j>0){// this loop is used to print numbers in a line
if(j>lines-i)
printf("*");
else
printf("%d",j);
j--;
}
if((lines-i)>9)
space=space+1;
printf("\n");
}
}
Output:
PHP Program:
<?php
$lines=15;
$i;
$j;
$k;
$l;
$space=0;
for($i=0;$i<$lines;$i++){// this loop is used to print lines
for($j=1;$j<=$space;$j++){//this loop is used to print space in a line
echo " ";
}
for($j=1;$j<=$lines;$j++){// this loop is used to print numbers
if($j<=($lines-$i))
echo $j;
else
echo "*";
}
$j--;
while($j>0){//this loop is used to print numbers in a line
if($j>$lines-$i)
echo "*";
else
echo $j;
$j--;
}
if(($lines-$i)>9)// this loop is used to increment space
$space=$space+1;
echo "<br>";
}
?>
Output:
Java Program:
public class Main
{
public static void main(String[] args) {
int lines=15;
int i,j,k,l;
int space=0;
for(i=0;i<lines;i++){// this loop is used to print lines
for(j=1;j<=space;j++){// this loop is used to print space in a line
System.out.print(" ");
}
for(j=1;j<=lines;j++){// this loop is used to print numbers in a line
if(j<=(lines-i))
System.out.print(j);
else
System.out.print("*");
}
j--;
while(j>0){// this loop is used to print numbers in a line
if(j>lines-i)
System.out.print("*");
else
System.out.print(j);
j--;
}
if((lines-i)>9)// this loop is used to increment space
space=space+1;
System.out.println("");
}
}
}
Output:
C# Program:
using System.IO;
using System;
public class Program
{
public static void Main(String[] args)
{
int lines=15;
int i,j,k,l;
int space=0;
for(i=0;i<lines;i++){// this loop is used to print the lines
for(j=1;j<=space;j++){// this loop is used to print space in a line
Console.Write(" ");
}
for(j=1;j<=lines;j++){// this loop is used to print numbers in a line
if(j<=(lines-i))
Console.Write(j);
else
Console.Write("*");
}
j--;
while(j>0){// this loop is used to print numbers in a line
if(j>lines-i)
Console.Write("*");
else
Console.Write(j);
j--;
}
if((lines-i)>9)// this loop is used to increment space
space=space+1;
Console.WriteLine("");
}
}
}
Output:
Python Program:
lines=15
i=1
j=1
k=1
l=1
space=0;
while i<lines: #this loop is used to print the lines
j=1
while j<=space:#this loop is used to print space in a line
print(' ', end='', flush=True)
j=j+1
j=1
while j<=lines:# this loop is used to print numbers in a line
if j<=lines-i:
print(j, end='', flush=True)
else:
print('*', end='', flush=True)
j=j+1
j=j-1
while j>0:# this loop is used to print numbers in a line
if j>lines-i:
print('*', end='', flush=True)
else:
print(j, end='', flush=True)
j=j-1
if lines-i>9:# this loop is used to increment space
space=space+1
print("")
i=i+1
C Program:
Output:
PHP Program:
Output:
Java Program:
Output:
C# Program:
Output:
Python Program:
Output:
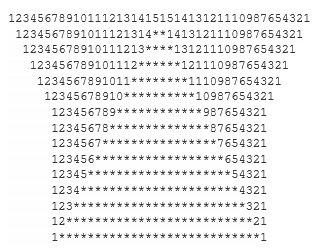
need an explanation for this answer? contact us directly to get an explanation for this answer