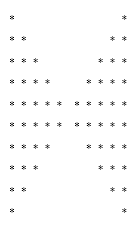
In this pattern program, we are going to draw above pattern on the output screen with the help of various loops.
Algorithm:
- STEP 1: START
- STEP 2: SET lines= 10
- STEP 3: SET space=(lines/2)-2
- STEP 4: SET i=1 REPEAT STEP 5 to STEP 17 UNTIL i is less than or equals to lines.
- STEP 5: SET flag1=0
SET l=1
- STEP 6: REPEAT STEP 7 and 8 UNTIL l is less than or equals to i
- STEP 7: IF flag1 is not true
PRINT '*' and INCREMENT flag1 by 1
ELSE PRINT BLANK SPACE "" WITH "*"
- STEP 8: SET l=l+1
- STEP 9: SET l=1
- STEP 10: REPEAT STEP 11 UNTIL l is less than or equals to space
- STEP 11: PRINT "" and SET l=l+1
- STEP 12: DECREMENT space by 4.
- STEP 13: SET l=1 and SET flag=1
- STEP 14: REPEAT STEP 15 UNTIL l is less than or equals to i
- STEP 15: IF flag is not true PRINT * and increment flag by 1.
ELSE PRINT BLANK SPACE "" WITH * .
- STEP 16: PRINT new line
- STEP 17: SET i=i+1
- STEP 18: INCREMENT space by 4
- STEP 19: SET i=lines/2
- STEP 20: REPEAT STEP 21 to STEP 25 UNTIL i is greater than 1
- STEP 21: SET flag=0 SET l=1
- STEP 22: REPEAT STEP 23 and 24 UNTIL l is less than or equals to i
- STEP 23: IF flag is not true PRINT *
ELSE PRINT "" + *
- STEP 24: SET l=l+1
- STEP 25: PRINT new line, SET i=i-1
- STEP 26: EXIT
C Program:
Output:
pattern
PHP Program:
Output:
Java Program:
Output:
C# Program:
Output:
Python Program:
Output:
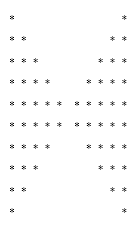
need an explanation for this answer? contact us directly to get an explanation for this answer