Explanation
In this program, we need to find the transpose of the given matrix and print the resulting matrix.
Transpose of a matrix:
Transpose of a matrix can be found by interchanging rows with the column that is, rows of the original matrix will become columns of the new matrix. Similarly, columns in the original matrix will become rows in the new matrix. The operation can be represented as follows:
[ AT ]ij = [ A ]ji
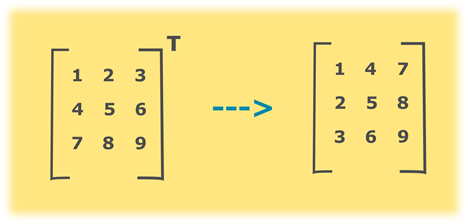
If the dimension of the original matrix is 2 × 3 then, the dimensions of the new transposed matrix will be 3 × 2.
Algorithm
- Declare and initialize a two-dimensional array a.
- Calculate the number of rows and columns present in the matrix and store it variables rows and cols respectively.
- Declare another array t with reversed dimensions i.e t[cols][rows]. Array t will be used to store the elements of the transposed matrix.
- Loop through the array a and convert its rows into columns of matrix t using
t[ i ][ j ] = a[ j ][ i ];
- Finally, display the elements of matrix t.
Input:
Matrix a = [1, 2, 3]
Output:
Transpose of given matrix: [1 4 7]
[2 5 8]
[3 6 9]
Python
Output:
C
Output:
JAVA
Output:
C#
Output:
PHP
Output: