Q:
How to fetch data from database in PHP and display in PDF
belongs to collection: PHP Programming Exercises
PHP Programming Exercises
- Write a php program to compare between things that are not integers
- Write a division table program in PHP using for loop
- Write a program in PHP to print prime numbers between 1 and 100
- Write a php program to print numbers from 10 to 1 using the recursion function
- Write a php program to store the username in cookie and check whether the user have successfully login or not
- Write a php program to convert the given string into an array
- Write a php program to loop over the json data
- Write a program in PHP to remove all html tags except paragraph and italics tags
- Write a program to loop through an associative array using foreach() or with each()
- Write a php program to differentiate between fgets, fgetss and fgetcsv
- There are two deals of an item to buy. The quantities and prices of the item are given below. Write a program in PHP to find the best deal to purchase the item
- Write a php program to set session on successful login
- Write a program in PHP to read from directory
- PHP create image from text and save
- How to get data from XML file in PHP
- PHP Create Word Document from HTML
- How to check whether a year is a leap year or not in PHP
- Fibonacci Series Program in PHP
- How to generate QR Code in PHP
- How does PHP store data in cache?
- How to detect a mobile device using PHP?
- How to send HTML form data to email using PHP?
- How to get location from IP address using PHP?
- How to lock a file using PHP?
- How to import a CSV file into MySQL using PHP
- How to fetch data from database in PHP and display in PDF
- How to insert image in database using PHP
- How to remove last character from string using PHP?
- Write a PHP program to reverse a string without predefined function
- Write a PHP program to calculate percentage of total
- How to sanitize input for MySQL using PHP?
- Write a program to calculate electricity bill in PHP
- How to send email with SMTP in PHP?
- How to Send Text Messages With PHP?
- How to convert stdClass object to Array in PHP?
- How do I import Excel data into MySQL database using PHP?
- How can I post a form without refreshing the page?
- How to sort table columns with PHP and MySQL?
- How to get current directory, filename and code line number in PHP
There are several PHP libraries available to generate PDF. In this exercise, we are using 'FPDF' to generate PDF. The F from FPDF stands for Free. This PHP library is used to generate PDF from UTF-8 encoded HTML. The FPDF contains high-level functions and is rich in features like image support, color support, page compression, automatic page break and link break. This library supports PHP version 5.1.
These are the steps to generate PDF to fetch data from database in PHP -
Download FPDF
Download the latest version of the FPDF library from its official website -
FPDF Library
Extract the zip file in your project directory.
Now, let's create a main PHP file 'index.php', that we will call in the browser. At the top of this page, include the FPDF library file.
require('fpdf/fpdf.php');
Make a Database Connection
Make sure to provide the right fpdf.php path on your main PHP file. After this, write the database connection code and make sure to replace 'hostname', 'username', 'password' and 'database' with your database credentials and name.
// Database Connection $conn = new mysqli('hostname', 'username', 'password', 'database'); //Check for connection error if($conn->connect_error){ die("Error in DB connection: ".$conn->connect_errno." : ".$conn->connect_error); }
FPDF Class
Next, instantiate the fpdf class -
$pdf = new FPDF();
The FPDF library has many pre-defined methods, we have used these methods among them.
AddPage() - To add a new page.
SetFont() - To set the font.
Cell() - To print a cell.
Ln() - Line break.
Complete Code: index.php
Here, we have merged all codes that were explained in detail above to generate PDF using the PHP FPDF library.
When, you will call this on the browser, it will look something like this -
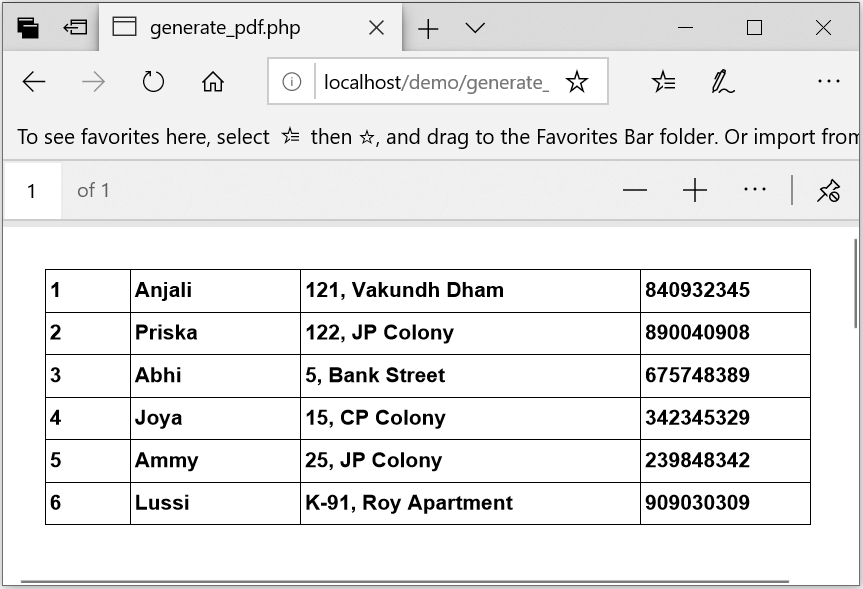
need an explanation for this answer? contact us directly to get an explanation for this answer