The spiral pattern (or matrix in spiral form) is frequently asked in Java interviews and academics. In this section, we will create a Java program to create a spiral pattern or spiral matrix.
What is Spiral Matrix or Spiral Pattern?
A spiral pattern is a number pattern that can be represented in matrix form. It is made up of a 2D array (m*n). Pattern may represent in two forms, either clockwise or anticlockwise.
In order to print a matrix in spiral pattern (clockwise) form, we need to follow the following traversal order:
- Left to right (first row)
- Top to bottom (Last column)
- Right to left (last row)
- Bottom to top (First column)
In order to print the spiral pattern (anticlockwise), reverse the above traversal order.
Let's understand the pictorial representation of the spiral patterns, as shown in the following figure.
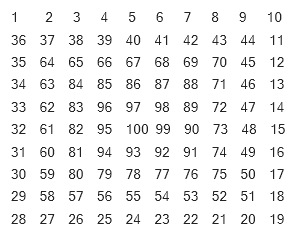
For example, consider the following 5*5 matrix:
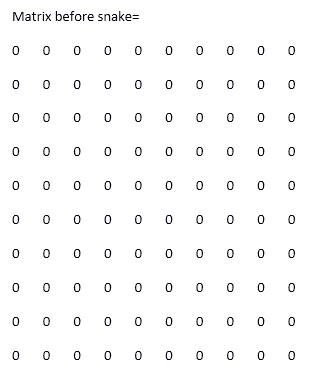
The above matrix can be represented in spiral form as follows:
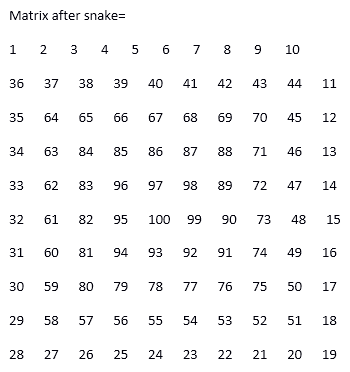
We can follow any of the approaches to print the spiral pattern:
Throughout this section, we have used the iterative approach.
What should be the approach?
- First, create a 2D array of size n.
- Declare the variables to store the boundary of the array. Also declare a variable to store the size left to print the spiral.
- For the movement over the array, use l, r, u, and d letters that represent the direction of movement left, right, up, and down, respectively.
- Execute a loop from 1 to n*2 to fill the array in spiral form.
Spiral Pattern Java Program
SpiralPaternExample1.java
Output:
Let's see another spiral pattern.
In the following program, we have used the following approach:
Starting the indexing from i = 1 and j = 1. It can be observed that every value of the required matrix will be max(abs(i - n), abs(j - n)) + 1.
SpiralPatternExample2.java
Output:
SpiralPatternExample3.java
Output:
Print a Given Matrix in Spiral Form
There are many approaches that can be used to print a matrix in spiral form.
SpiralToMatrixExample.java
Output: