In this section, we will learn what is a spy number and also create Java programs to check if the given number is Spy or not. The spy number program is frequently asked in Java coding test.
Spy Number
A positive integer is called a spy number if the sum and product of its digits are equal. In other words, a number whose sum and product of all digits are equal is called a spy number.
Example of Spy Number
Let's take the number 1124 and check whether the number is a spy or not. First, we will split it into digits (1, 1, 2, 4). After that find the sum and product of all the digits.
Sum=1+1+2+4=8
Product=1*1*2*4=8
We observe that the sum and product of the digits both are equal. Hence, 1124 is a spy number.
Similarly, we can check other numbers also. Some other spy numbers are 22, 123, 132, etc.
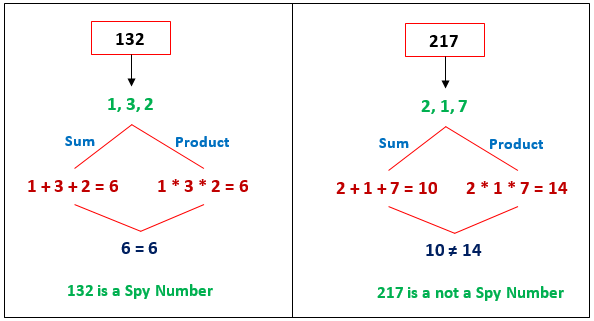
Steps to Find Spy Number
- Read or initialize a number (n) that you want to check.
- Declare two variables sum and product to store sum and product of digits. Initialize sum with 0 and product with 1.
- Find the last digit (n%10) of the given number by using the modulo operator.
- Add the last digit to the variable sum.
- Multiply the last digit with the product variable.
- Divide the given number (n) by 10. It removes the last digit.
- Repeat steps 3 to 6 until the given number (n) becomes 0.
- If the variable sum and product have the same value, then the given number (n) is a spy number, else not a spy number.
Spy Number Java Program
SpyNumberExample1.java
Output 1:
Output 2:
SpyNumberExample2.java
Output: