#include <stdio.h>
int matrix[10][10];
int main()
{
int i,j,k,l;
int direction=1;
printf("Matrix before snake=\n");
for(i=0;i<10;i++){ //this loop is used to print one line of a matrix
for(j=0;j<10;j++){// this loop is used to print one element in a line
printf("%d\t",matrix[i][j]);
}
printf("\n");
}
for(i=1,j=0,k=0;i<=100;i++){ /*this is loop is used to set martixpattern */
matrix[j][k]=i;
switch(direction){ //switch is used to check direction of snake
case 1:if(k+1<10){
if(matrix[j][k+1]==0){
k++; //if direction is one and martix[j][k+1] is 0
continue;
}
else{
j++;//if direction is one but k+1 is not smaller than 10
direction=2;
continue;
}
}
else{ //if direction is one and martix[j][k+1] is 0
j++;
direction=2;
continue;
}
break;
case 2:if(j+1<10){
if(matrix[j+1][k]==0){ /* if direction=2 and matrix[j+1][k]=0 */
j++;
continue;
}
else{
direction=3;
k--; // if matrix[j+1][k] is not equal 0
continue;
}
}
else{// if j+1 is not less than 10
direction=3;
k--;
continue;
}
break;
case 3:if(k-1>=0){
if(matrix[j][k-1]==0){
k--; // if direction=3 and matrix[j][k-1]=0
continue;
}
else{
direction=4;
j--; //if matrix[j][k-1] is not equals to zero
continue;
}
}
else{
direction=4;
j--;// k-1 is not greater than -0
continue;
}
break;
case 4:if(j-1>=0){
if(matrix[j-1][k]==0){
j--; //if direction=4 and matrix[j-1][k]=0
continue;
}
else{
k++; // if direction=4 and matrix[j-1][k]!=0
direction=1;
continue;
}
}
else{
k++; // if direction=4 but j-1 is not greater than -1
direction=1;
continue;
}
break;
}
}
printf("Matrix after snake=\n");
for(i=0;i<10;i++){ // this loop is used to print one line of matrix
for(j=0;j<10;j++){ // this loop is used to print one element of line
printf("%d\t",matrix[i][j]);
}
printf("\n");
}
return 0;
}
Output:
PHP Program:
<?php
$i;
$j;
$k;
$l;
$direction=1;
$matrix=array();
for($i=0;$i<10;$i++){// this loop is used to access one line of matrix
for($j=0;$j<10;$j++){// this loop is used to access one element
$matrix[$i][$j]=0;
}
}
echo "Matrix before snake=";
echo "<br>";
for($i=0;$i<10;$i++){// this loop is used to access one line of matrix
for($j=0;$j<10;$j++){ // this loop is used to access one element
echo $matrix[$i][$j];
echo " ";
}
echo "<br>";
}
for($i=1,$j=0,$k=0;$i<=100;$i++){// this loop is used set pattern
$matrix[$j][$k]=$i;
switch($direction){// this switch is used to switch the direction
case 1:if($k+1<10){
if($matrix[$j][$k+1]==0){
$k++;// if direction=1 and matrix[j][k+1]=0
continue;
}
else{
$j++;// if direction=1 and matrix[j][k+1]!=0
$direction=2;
continue;
}
}
else{ //if k+1 is not smaller than 10
$j++;
$direction=2;
continue;
}
break;
case 2:if($j+1<10){
if($matrix[$j+1][$k]==0){
$j++; // if direction=2 and matrix[j+1][k]=0
continue;
}
else{
$direction=3;
$k--; //if direction=2 and matrix[j+1][k]!=0
continue;
}
}
else{
$direction=3;
$k--;// if j+1 is not smaller than 10
continue;
}
break;
case 3:if($k-1>=0){
if($matrix[$j][$k-1]==0){
$k--; // if direction=3 and matrix[j][k-1]=0
continue;
}
else{
$direction=4;
$j--; // if direction=3 and matrix[j][k-1]!=0
continue;
}
}
else{
$direction=4;
$j--; // if k-1 is not greater than -1
continue;
}
break;
case 4:if($j-1>=0){
if($matrix[$j-1][$k]==0){
$j--; // if direction=4 and matrix[j-1][k]=0
continue;
}
else{
$k++; // if direction=4 and matrix[j-1][k]!=0
$direction=1;
continue;
}
}
else{
$k++; //if j-1 is not greater than -1
$direction=1;
continue;
}
break;
}
}
echo "Matrix after snake";
echo "<br>";
for($i=0;$i<10;$i++){ // this loop is used to print one line
for($j=0;$j<10;$j++){ //this loop is used to print one element
echo $matrix[$i][$j];
echo " ";
if($matrix[$i][$j]<100){
echo " ";
}
if ($matrix[$i][$j]<10){
echo " ";
}
}
echo "<br>";
}
Output:
Java Program:
public class pattern{
public static void main(String []args){
int matrix[][]=new int[10][10];
int i,j,k,l;
int direction=1;
for(i=0;i<10;i++){ //this loop is used to access one line of matrix
for(j=0;j<10;j++){ // this loop is used to access one element
matrix[i][j]=0;
}
}
System.out.print("Matrix before snake=\n");
for(i=0;i<10;i++){ // this loop is used to print one line of matrix
for(j=0;j<10;j++){//this loop is used to print one element of matrix
System.out.print(matrix[i][j]+"\t");
}
System.out.println("");
}
for(i=1,j=0,k=0;i<=100;i++){// this loop is used to set pattern
matrix[j][k]=i;
switch(direction){
case 1:if(k+1<10){
if(matrix[j][k+1]==0){
k++; // if direction=1 and matrix[j][k+1]=0
continue;
}
else{
j++; // if direction=1 and matrix[j][k+1]!=0
direction=2;
continue;
}
}
else{
j++; // if k+1 is not smaller than 10
direction=2;
continue;
}
case 2:if(j+1<10){
if(matrix[j+1][k]==0){
j++; //if direction=2 and matrix[j+1][k]=0
continue;
}
else{
direction=3;
k--; //if direction=2 and matrix[j+1][k]!=0
continue;
}
}
else{
direction=3;
k--; //j+1 is not smaller than 10
continue;
}
case 3:if(k-1>=0){
if(matrix[j][k-1]==0){
k--; // if direction=3 and matrix[j][k-1]=0
continue;
}
else{
direction=4;
j--; // if direction=3 and matrix[j][k-1]!=0
continue;
}
}
else{
direction=4;
j--; //k-1 is not greater than -1
continue;
}
case 4:if(j-1>=0){
if(matrix[j-1][k]==0){
j--; //if direction=4 and matrix[j-1][k]=0
continue;
}
else{
k++; //if direction=4 and matrix[j-1][k]!=0
direction=1;
continue;
}
}
else{
k++; //if j-1 is not greater than -1
direction=1;
continue;
}
}
}
System.out.println("Matrix after snake=\n");
for(i=0;i<10;i++){ //this loop is used to print one line of matrix
for(j=0;j<10;j++){ //this loop is used to print one element
System.out.print(matrix[i][j]+"\t");
}
System.out.println("");
}
}
}
Output:
C# Program:
using System.IO;
using System;
public class Program
{
public static void Main(String[] args)
{
int[,] matrix=new int[10,10];
int i,j,k;
int direction=1;
for(i=0;i<10;i++){ // this loop is used to access one line of a matrix
for(j=0;j<10;j++){ //this loop is used to access one element
matrix[i,j]=0;
}
}
Console.WriteLine("Matrix before snake=\n");
for(i=0;i<10;i++){ // this loop is used to print one line of matrix
for(j=0;j<10;j++){ // this loop is used to print one element
Console.Write(matrix[i,j]+"\t");
}
Console.WriteLine("\n");
}
for(i=1,j=0,k=0;i<=100;i++){// this loop is used to set pattern
matrix[j,k]=i;
switch(direction){
case 1:if(k+1<10){
if(matrix[j,k+1]==0){
k++; //if direction=1 and matrix[j][k+1]=0
continue;
}
else{
j++; //if direction=1 and matrix[j][k+1]!=0
direction=2;
continue;
}
}
else{
j++; //if k+1 is not smaller than 10
direction=2;
continue;
}
case 2:if(j+1<10){
if(matrix[j+1,k]==0){
j++; //direction=2 and matrix[j+1][k]=0
continue;
}
else{
direction=3;
k--; //direction=2 and matrix[j+1][k]!=0
continue;
}
}
else{
direction=3;
k--; //j+1 is not smaller than 10
continue;
}
case 3:if(k-1>=0){
if(matrix[j,k-1]==0){
k--; // if direction=3 and matrix[j][k-1]=0
continue;
}
else{
direction=4;
j--; // if direction=3 and matrix[j][k-1]!=0
continue;
}
}
else{
direction=4;
j--; //if k-1 is not greater than -1
continue;
}
case 4:if(j-1>=0){
if(matrix[j-1,k]==0){
j--; //if direction=4 and matrix[j-1][k]=0
continue;
}
else{
k++; //if direction=4 and matrix[j-1][k]!=0
direction=1;
continue;
}
}
else{
k++; //if j-1 is not greater than -1
direction=1;
continue;
}
}
}
Console.WriteLine("Matrix after snake=\n");
for(i=0;i<10;i++){ // this loop is used to print one line
for(j=0;j<10;j++){ // this loop is used to print one element
Console.Write(matrix[i,j]+"\t");
}
Console.WriteLine("\n");
}
}
}
Output:
Python Program:
i=0
j=0
k=1
l=1
direction=1
matrix=[[0,0,0,0,0,0,0,0,0,0],[0,0,0,0,0,0,0,0,0,0],[0,0,0,0,0,0,0,0,0,0],[0,0,0,0,0,0,0,0,0,0],[0,0,0,0,0,0,0,0,0,0],[0,0,0,0,0,0,0,0,0,0],[0,0,0,0,0,0,0,0,0,0],[0,0,0,0,0,0,0,0,0,0],[0,0,0,0,0,0,0,0,0,0],[0,0,0,0,0,0,0,0,0,0]]
print("Matrix before snake=")
i=0
while i<10: #this loop is used to print one line of matrix
j=0
while j<10: #this loop is used to print one element
print(matrix[i][j], end='', flush=True)
print(" ", end='', flush=True)
if matrix[i][j]<100:
print(" ", end='', flush=True)
if matrix[i][j]<10:
print(" ", end='', flush=True)
j=j+1
print("")
i=i+1
i=1
j=0
k=0
while i<=100: #this loop is used to set pattern
matrix[j][k]=i
if direction==1:
if k+1<10:
if matrix[j][k+1]==0:
k=k+1 #if direction=1 and matrix[j][k+1]=0
else:
j=j+1 #if direction=1 and matrix[j][k+1]!=0
direction=2
else:
j=j+1 #if k+1 is not smaller than 10
direction=2
elif direction==2:
if j+1<10:
if matrix[j+1][k]==0:
j=j+1 #if direction=2 and matrix[j+1][k]=0
else:
direction=3
k=k-1 #if direction=2 and matrix[j+1][k]!=0
else:
direction=3
k=k-1 #if j+1 is not smaller than 10
elif direction==3:
if k-1>=0:
if matrix[j][k-1]==0:
k=k-1 #if direction=3 and matrix[j][k-1]=0
else:
direction=4
j=j-1 #if direction=3 and matrix[j][k-1]!=0
else:
direction=4
j=j-1 #if k-1 is not greater than -1
elif direction==4:
if j-1>=0:
if matrix[j-1][k]==0:
j=j-1 #if direction=4 and matrix[j-1][k]=0
else:
k=k+1 #if direction=4 and matrix[j-1][k]!=0
direction=1
else:
k=k+1 #if j-1 is not greater than -1
direction=1
i=i+1
print("Matrix after snake=")
i=0
while i<10: #this loop is used to print one line
j=0
while j<10: #this loop is used to print one element
print(matrix[i][j], end='', flush=True)
print(" ", end='', flush=True)
if matrix[i][j]<100:
print(" ", end='', flush=True)
if matrix[i][j]<10:
print(" ", end='', flush=True)
j=j+1
print("")
i=i+1
C Program:
Output:
PHP Program:
Output:
Java Program:
Output:
C# Program:
Output:
Python Program:
Output:
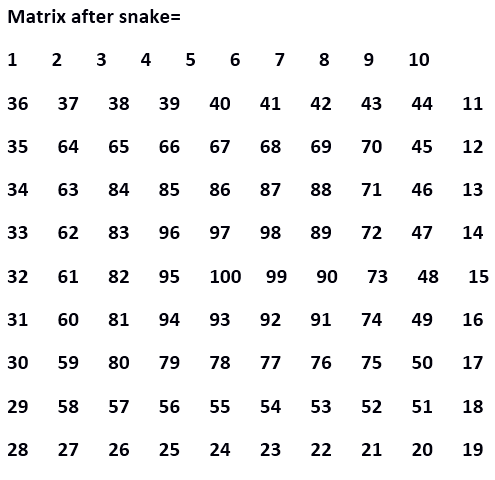
need an explanation for this answer? contact us directly to get an explanation for this answer