Obeying the following rules:
- Only one disk can be transfer at a time.
- Each move consists of taking the upper disk from one of the peg and placing it on the top of another peg i.e. a disk can only be moved if it is the uppermost disk of the peg.
- Never a larger disk is placed on a smaller disk during the transfer.
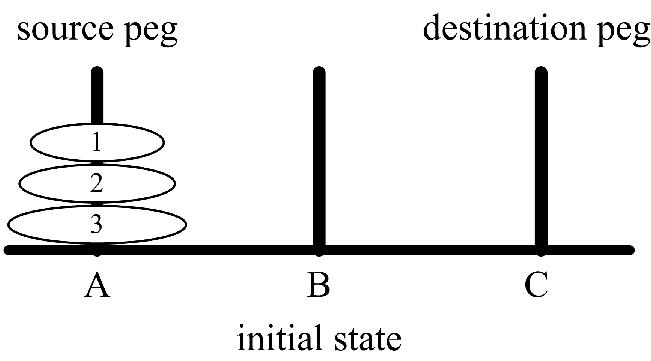
(figure 1)
The solution to the puzzle calls for an application of recursive functions and recurrence relations.
A skeletal recursive procedure (Outline) for the solution of the problem for N number of disks is as follows:
- Move the top N-1 disks from peg A to peg B (using C as an auxiliarypeg)
- Move the bottom disk from peg A to peg C
- Move N-1 disks from Peg B to Peg C (using Peg A as an auxiliary peg)
The pictorial representation of the skeletal recursive procedure for N=4 disks is shown in Figure 2.
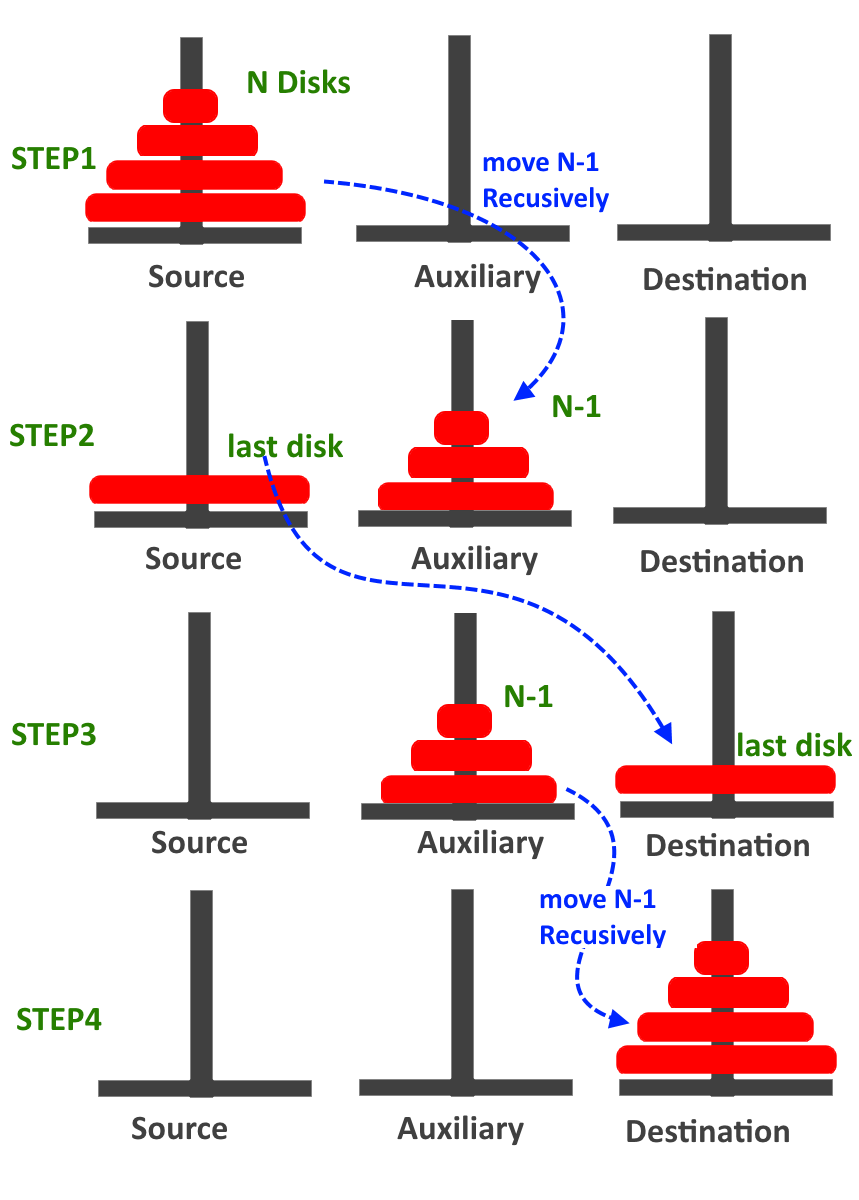
Algorithm
Let’s take an example to better understand the algorithm (For n=3).
(figure 3)
Implementation of Tower of HANOI in using C++ program
Output