Given Preorder and Inorder traversal of the tree
For Example: A tree is given
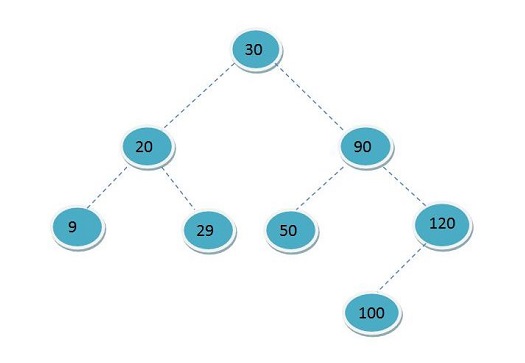
Inorder traversal of the given tree is: - 9, 20, 29, 30, 50, 90, 100, 120
Preorder traversal of the given tree is: - 30, 20, 9, 29, 90, 50, 120, 100
Postorder traversal of the given tree is: - 9, 29, 20, 50, 100, 120, 90, 30
Algorithm:
As we know that in preorder traversal root is always the first element so we will find the left and right subtree recursively and at last the root, for that we have to search inorder for the left and right subtree. First we will search elements of preorder in inorder as the elements after the index of the searched element in inorder are the members of the right subtree and elements before the index are elements of right subtree and the searched element is the root of that subtree.
Consider the program:
Output