belongs to collection: C Programs to print Series
In this exercise, I will show you, How to write a C program to print hollow square star pattern with diagonal. Here, one thing is important to know that all sides of the square must be the same.
#include <stdio.h> int main() { int x = 0,y = 0; unsigned int squareSide = 0; printf("Enter Side of a Square = "); scanf("%u",&squareSide); for(x=1; x<=squareSide; ++x) { for(y=1; y<=squareSide; ++y) { // Checking boundary conditions and main // diagonal and secondary diagonal conditions if((x==1) || (x==squareSide) || (y==1) || (y==squareSide) || (x==y)|| (y==(squareSide - x + 1))) { //Print star printf("*"); } else { //Print space printf(" "); } } // Print new line printf("\n"); } return 0; }
Output:
---------------------------------------------------------------------------------------------------------------
#include <stdio.h> #define isBoundary(x,y,n) ((x==1) || (x==n) || (y==1) || (y==n) || (x==y)|| (y==(n - x + 1))) int main() { int x = 0,y = 0; unsigned int squareSide = 0; printf("Enter Side of a Square = "); scanf("%u",&squareSide); for(x=1; x<=squareSide; ++x) { for(y=1; y<=squareSide; ++y) { isBoundary(x,y,squareSide)? printf("*"):printf(" "); } // Print new line printf("\n"); } return 0; }
total answers (1)
start bookmarking useful questions and collections and save it into your own study-lists, login now to start creating your own collections.
Method1:
Output:
---------------------------------------------------------------------------------------------------------------
Method2:
Output:
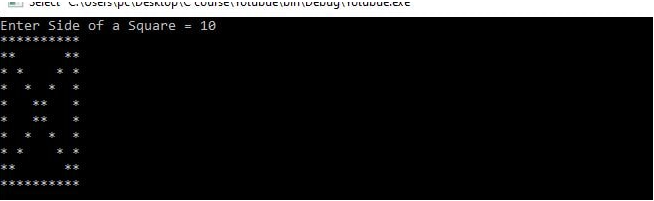
need an explanation for this answer? contact us directly to get an explanation for this answer