We can create a java program to sort array elements using selection sort. In selection sort algorithm, we search for the lowest element and arrange it to the proper location. We swap the current element with the next lowest number.
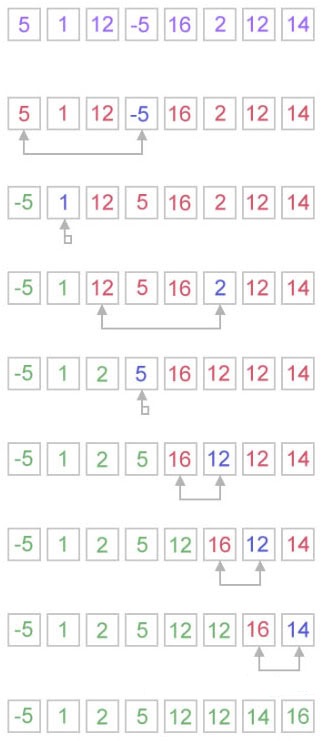
How does selection sort work?
The selection sort algorithm works in a very simple way. It maintains two subarray for the given array.
- The subarray is already sorted.
- And the second subarray is unsorted.
With every iteration of selection sort, an element is picked from the unsorted subarray and moved to the sorted subarray.
arr[] = 25 35 45 12 65 10
// Find the minimum element in arr[0...5] and place it at beginning.
10 25 35 45 12 65
// Find the minimum element in arr[1...5] and place it at beginning of arr[1...5]
10 12 25 35 45 65
// Find the minimum element in arr[2...5] and place it at beginning of arr[2...5]
No, you can see that the array is already sorted.
10 12 25 35 45 65
Time Complexity
Best: ?(n^2)
Average: ?(n^2)
Worst: O(n^2)
Space Complexity
O(1)
Selection Sort Java Example
Output:
Selection Sort in Java (Another way)
You can also use a method where array is not predefined. Here, user has to put the elements as input.
In the following Java program, we ask user to enter the array elements or number, now compare the array's element and start swapping with the variable temp. Put the first element in the temp and the second element in the first, and then temp in the second number and continue for the next match to sort the whole array in ascending order.
Output:
Use image SelectionSort
need an explanation for this answer? contact us directly to get an explanation for this answer