In this article, we will see the programs to find the area and perimeter of the trapezium in different programming languages.
But before writing the programs, let's first see a brief description of the trapezium and the formulae to find its area and perimeter.
Trapezium
The trapezium (also called trapezoid) is a geometrical shape that includes four sides in which a pair of opposite sides is parallel. The parallel sides are known as base, and the non-parallel sides are known as leg. The parallel sides can be horizontal, vertical, or slanting (diagonal).
It is a convex quadrilateral shape. It means that a closed shape with four sides along with one pair of parallel sides.
The representation of the trapezium is shown in the below image -
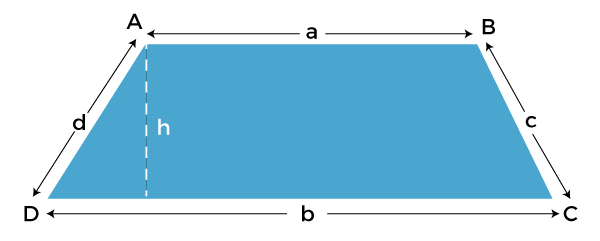
Area of trapezium
The formula for finding the area of the trapezium are given as follows -
Area of trapezium (A) = ½ (b1 + b2) * h
Or,
Area of trapezium (A) = h/2 * (b1 + b2)
Or,
Area of trapezium (A) = h * (b1 + b2/2)
Perimeter of trapezium
The perimeter of a trapezium is the sum of all its sides. The formula for finding the perimeter of the trapezium with sides AB, BC, CD, and DA are given as follows -
Perimeter of trapezium = Sum of all sides = AB + BC + CD + DA
If AB = a, BC = b, CD = c, DA = d, the perimeter of trapezium will be -
Perimeter of trapezium = Sum of all sides = a + b + c + d
Now, let's see the programs to find the area and perimeter of the trapezium.
Programs to find the area and perimeter of trapezium
In the programs, we are using the following formulae to calculate the area and perimeter of trapezium -
Area of trapezium = ½ * (a + b) * h (Where, 'a' and 'b' are the parallel sides of trapezium, and 'h' is the perpendicular distance between parallel sides)
Perimeter of trapezium = a + b + c + d (Where, 'a', 'b', 'c', and 'd' are the sides of trapezium)
Program: Write a program to find the area and perimeter of the trapezium in C language.
Output:
Program: Write a program to find the area and perimeter of the trapezium in the C# language.
Output:
Program: Write a program to find the area and perimeter of the trapezium in Java.
Output:
After compiling the file on command prompt, and after execution, the output will be -
Program: Write a program to find the area and perimeter of trapezium in JavaScript.
Output:
Program: Write a program to find the area and perimeter of the trapezium in C++.
Output:
Program: Write a program to find the area and perimeter of trapezium in PHP.
Output:
Program: Write a program to find the area and perimeter of trapezium in python.
Output:
So, that's all about the article. Here, we have discussed the programs to find the area and perimeter of the trapezium in C, C++, Java, C#, PHP, python, and JavaScript. Hope you find the article helpful and informative.
need an explanation for this answer? contact us directly to get an explanation for this answer