A C# code was implemented to calculate a student’s GPA. The requirements were considered before writing the code are:
- Module credit can not be negative.
- The maximum module credit is 10.
- The total number of credits a student can take is 18.
- The maximum number of modules a student can take is 10.
- The program must ask a user if they want to calculate another GPA and only accept ”n” or ”y” (”n” for no and ”y” for yes).
- The maximum module grade is 100.
- The minimum module grade is 0.
- The program must convert the module grade to point based on Table 1.
- The total input module credits should be at least 1
Table 1: The equivalent point for each grade
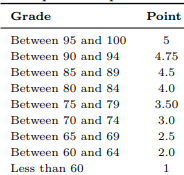
You have to test the provided code and check whether it meets the above requirements or not.
Submission instructions
2.1 Run files
When you run the code in the GPA.cs file (it can be downloaded from the Blackboard), the input you entered will be saved to a file called ”run No.”, for example, run 1. The number in the file name will be increased in each run.
2.2 Test cases template
You have to submit the input that you used to test each requirement and explain the expected output and what was the actual output.
2.2.1 Test case example
Consider the following requirement:
• The program must not accept the same module more than once.
We may use the following input to test if the code will accept the same module name again or not.
2
CIT420
4
90
CIT420
4
80
n
And the output of the code was:
Module name Module credit Module point
CIT420 4 19
CIT420 4 16
GPA: 4.375
The code saved the input and the output to the file called run 6.sh and output 6.sh, respectively. It is clear from the input and the output that the code does not meet this requirement and accepts the same module name twice. We can report this test case using the following template.
Requirement |
Test input |
Test actual output |
Test expected output |
The program must not accept the same module more than once. |
run 6.sh |
output 6.sh |
We expected an error message when the same module name uses again. |
note: this solution is not complete 100%, there are som mistakes in it
GPATest.cs
GPA.cs
need an explanation for this answer? contact us directly to get an explanation for this answer