Q1) Study the following Class diagrams implemented in BlueJ IDE. The classes are:
1. EMP
2. DEPT
3. ORG
Apply the following tasks:
A. Write the implementation for the classes (EMP & DEPT). [Fields, Constructors, Methods].
B. ORG class is the main class.
C. Apply the following Object-Oriented Concepts through the implementation of the classes:
Ø Overloading Constructors. ( multiple constructors)
Ø Accessor Methods.
Ø Mutator Methods.
Ø Internal Method call.
Ø External Method call.
Ø Variables Scope.
Ø Creating Objects of one class inside other class.{ objects of EMP and DEPT must be created inside the main class ORG}.
Q2) Create a collection inside the class ORG, the collection must contains objects of class EMP. Apply the following actions:
Ø Add 7 objects of EMP.
Ø Remove the object at index 3
Ø Print out all the objects
Ø Check whether the collection is empty or not.
Ø Replace objects 3 with object 1.
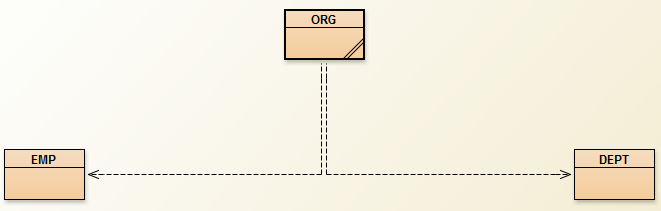
need an explanation for this answer? contact us directly to get an explanation for this answer