Implementing a Round Robin Scheduling Algorithm in C++ programming language with a Gantt chart and arrival time. As we all know the Round Robin CPU Scheduling Algorithm, so we have to Write a Program code In C++ language to check how it performs all the operations. We are also going to discuss the Turn around time, burst time and execution time. In this article, you will get all the knowledge about the Round-robin. How it's works and the pro and cons.
Algorithms Explanation
We can Understand Round Robin Scheduling Algorithm by taking an example Suppose there is 4 process. and each process comes at the same time so based on FIFO(First in First Out) scheduler keep all process in Ready Queue and forgiven time Slice each process will be executed until all process finish. Let's take an example and try to understand How Round Robin Works.
Round Robin Example
Quantum = 4
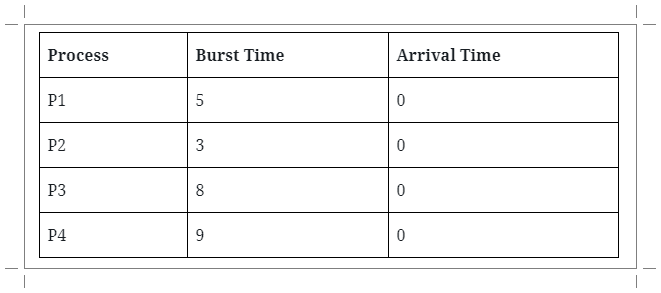
Now Schedular keeps all process in Ready Queue and based on FIFO(First in First Out) send the first process for execution.
P1 P2 P3 P4 P1 P3 P4 P4
0 4 7 11 12 16 20 21
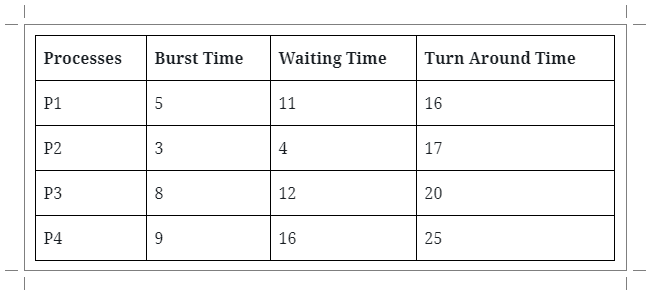
Average waiting time = 10.75
Average turn around time = 17
What is Round Robin Scheduling Algorithm?.
Round Robin is a primitive Scheduling Algorithm and most important and commonly used scheduling algorithm for CPU. In Round Robin Scheduling Algorithm each process has its own execution time that is called "Quantum".
After Quantum time next process start executes for given Quantum time and so on once a cycle complete again process execution start from first, process and repeat the process again and again and for saving a current state of process Context switching is used.
- -Round Robin is a primitive Scheduling Algorithm.
- -Round Robin follow FIFO(First in First Out) Principle.
- -For executing each process in Round Robin Time cluster or time Slice provides, so a process can execute for a particularly given amount of time, the given time is called Quantum.
- -After Quantum time for saving a state of each process Context switching is used.
- -Round Robin is Great to use for fully Utilization of a CPU and Multitasking.
- -Scheduler always needs to keep ready next process ready in ready Queue or Queue for execution in CPU so we can say that scheduler play an important role in the round-robin.
- -After Quantum Time for each process, the same step repeats again and again.
How to Calculate Turn Around Time?.
Turn Around Time = Completion Time – Arrival Time, With the help of this formula, we can calculate a Turn Around Time of all process in Queue.
How to Calculate Waiting Time?.
Waiting Time = Turn Around Time – Burst Time, This formula is used for calculating the waiting time for the rest of the process.
Output:
Enter the Total Number of Process: 5
Enter an Arrival Time of the Process P1: 3
Enter an Arrival Time of the Process P2: 2
Enter an Arrival Time of the Process P3: 1
Enter an Arrival Time of the Process P4: 5
Enter an Arrival Time of the Process P5: 2
Enter a Burst Time of the Process P1: 1
Enter a Burst Time of the Process P2: 2
Enter a Burst Time of the Process P3: 4
Enter a Burst Time of the Process P4: 5
Enter a Burst Time of the Process P5: 2
Enter the Time Slice or Quantum: 3
=====================================================
Here AT = Arrival Time
BT = Burst Time
TAT = Turn Around Time
WT = Waiting Time
===================TABLE==============================
Process AT BT FT TAT WT
P1 3 1 4 1.000000 0.000000
P2 2 2 6 4.000000 2.000000
P3 1 4 15 14.000000 10.000000
P4 5 5 17 12.000000 7.000000
P5 2 2 14 12.000000 10.000000
=====================================================
Average Turn Around Time: 8.600000
Average Waiting Time: 5.800000
need an explanation for this answer? contact us directly to get an explanation for this answer