convert the following uml class diagram to equavalent java programming code:
in this example we will use most of OOP features in java programming, like:
- methods
- class and object
- instance and constructor
- static and non static
- access modifiers ( public, private, default, protected).
- encapsulation (set and get)
- inheritence
- method overriding
- arrays of objects
- polymorphism
----------------------------------------------------
develop a simple HR information system to manage employees salaries,
any employee has basic properties like: id, name, born date, gender, basic salary, accomodation allowance, food allowance
there are 2 types of employees:
this employee gets his salary upon hourly basis, the normal work hours in the month are 174 hours, any extra work hours will be added to basic salary.
this employee gets his salary without calculation of worked hours, and takes a monthly bonus added to his basice salary, the bonus is calculated based on employee classification ( class 1 for managers: bonus is 20% out of basic salary, class 2 for moderate managers: bonus is 15% out of basic salary, class 3 for operators: bonus is 10% out of basic salary)
----------------------------------
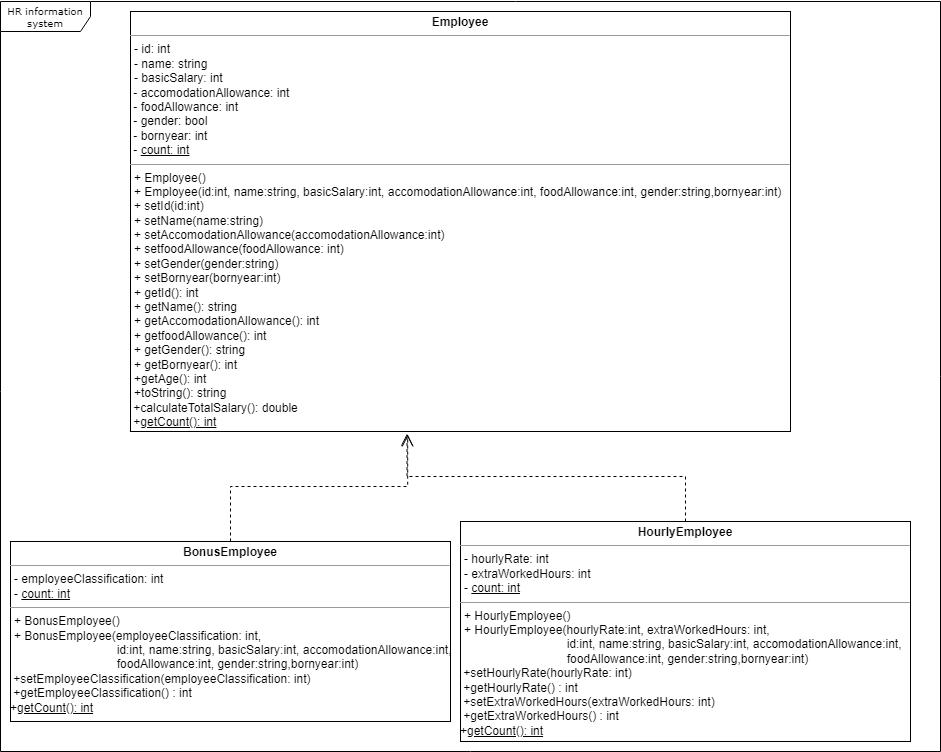