Fibonacci series is the one in which you will get your next term by adding previous two numbers.
For example,
0 1 1 2 3 5 8 13 21 34
Here, 0 + 1 = 1
1 + 1 = 2
3 + 2 = 5
and so on.
Logic:
- Initializing first and second number as 0 and 1.
- Print first and second number.
- From next number, start your loop. So third number will be the sum of the first two numbers.
Example:
We'll show an example to print the first 12 numbers of a Fibonacci series.
Output:
Fibonacci series using Recursive function
Recursion is a phenomenon in which the recursion function calls itself until the base condition is reached.
Output:
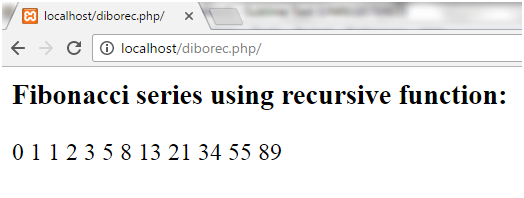
need an explanation for this answer? contact us directly to get an explanation for this answer