The pyramid is like a triangular structure. Pyramid programs are used to extend coding, thinking, logic, looping and nested looping concepts. The interviewer usually asks these patterns to examine the logic and programming skills of the interviewee. In this topic, we will learn how to create various pyramid patterns in Visual Basic.
1. Star Pattern Programs
Program to print the Half Pyramid
Module1.vb
Output:
Program to print an inverted half pyramid
Module2.vb
Output:
Program to print an inverted full pyramid
Module3.vb
Output:
Program to print the full pyramid
Module4.vb
Output:
Program to print two half pyramids
Module5.vb
Output:
Program to print a pyramid shape
Module6.vb
Output:
Program to print a full pyramid
Module7.vb
Output:
Program to print a right inverted pyramid
Module8.vb
Output:
Program to print a right pyramid
Module9.vb
Output:
2. Number Pattern Program
Program to print the Half Pyramid of number
Module1.vb
Output:
Program to print the Floyd's Triangle
Module2.vb
Output:
Program to print the Half Pyramid of the same number
Module3.vb
Output:
Program to print an inverted half pyramid of number
Module4.vb
Output:
Program to print an inverted half pyramid of numbers
Module5.vb
Output
Program to print the Pascal's triangle
Module6.vb
Output:
3. Alphabets Pattern Program
Program to print a half pyramid of alphabets
Module1.vb
Output:
Program to print a half pyramid of alphabets
Module2.vb
Output:
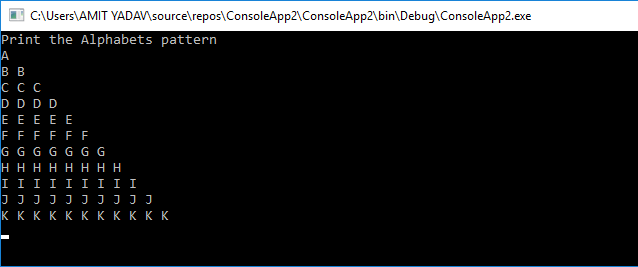
need an explanation for this answer? contact us directly to get an explanation for this answer