We are going to write a java calculator program using AWT(Abstract Window Toolkit) and applet. As we know that calculator program should perform some basics operation such as Addition, Subtraction, Multiplication, and division using Plus, Minus, Multiply and Division operators present in our keyboard. Calculator program in java using AWT and applet is based on GUI(Graphical User Interface).
Java AWT Calculator Operations and Task
There are four operations needs to perform a GUI(
Graphical User Interface) based calculator, operations or tasks are follow.
- Addition
- Subtraction
- Multiplication
- division
The list of operation of the task will be performed by the total 16 buttons and one text view area or result view window. These sixteens buttons are ten(0-9) number buttons, four operator buttons, one dot buttons and one equal button.
How to Run Java Applet Program
First, you need to download or copy-paste the program to any text editor you have, after that you need to save the program as "
calculator.java" if you wish to change the program name you need to make a changes in two more places one is the applet code and the second one is the class name in java program. If you do not make any changes as suggested in the below you will get an error "
applet is not initialized". Both are shown in the below example.
Output:
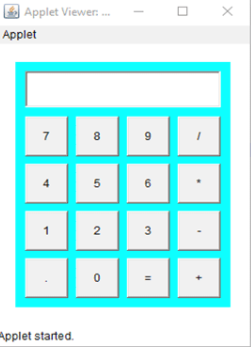
need an explanation for this answer? contact us directly to get an explanation for this answer